How to Use the WordPress Block Editor (Gutenberg)
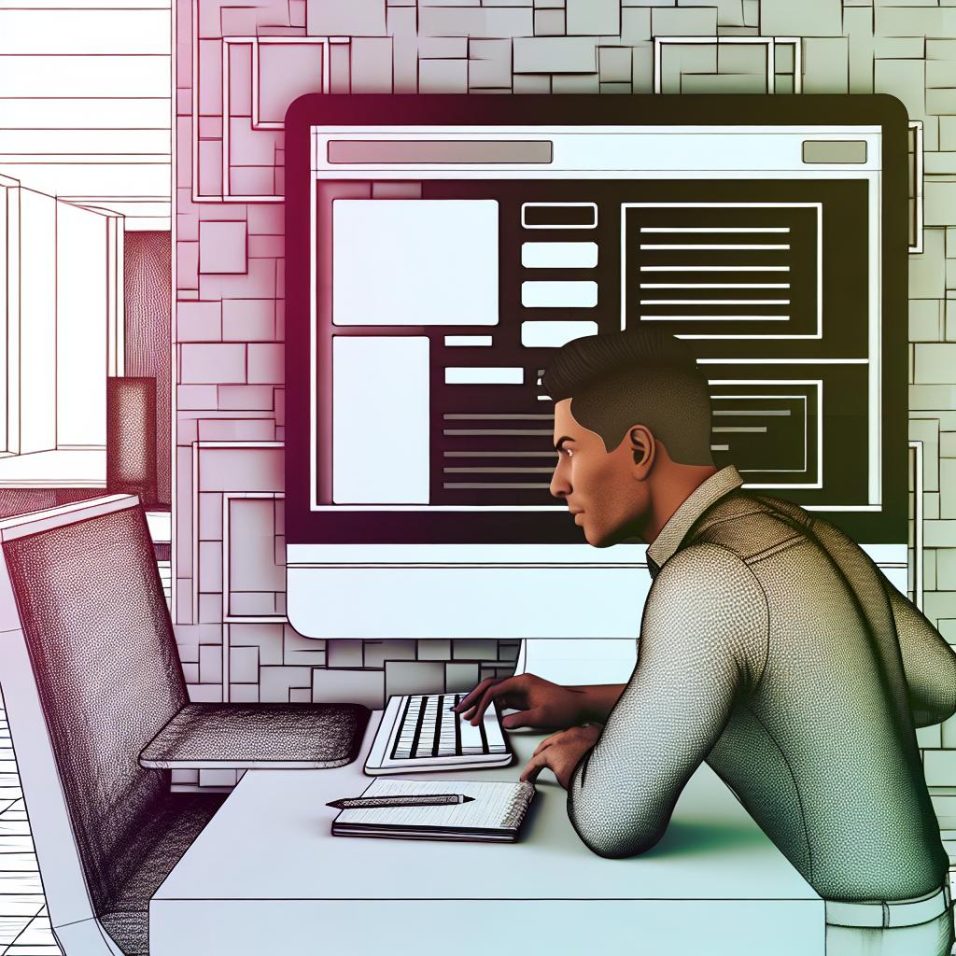
The WordPress Block Editor, also known as Gutenberg, is a transformative tool designed to enhance content creation on WordPress. It is essential for WordPress bloggers to master this editor to produce high-quality content. To start using the Block Editor, simply create a new post or page to access its intuitive interface, which allows you to add diverse blocks to structure your content.
Blocks are fundamental to Gutenberg, with each block representing an individual piece of content such as a paragraph, image, or video. These blocks can be easily added, rearranged, or deleted. Adding a block is straightforward: click the ‘+’ icon or type ‘/’ followed by the block name within a paragraph block (e.g., ‘/image’ for an image block).
Each block has specific settings accessible via the right sidebar, allowing customization like alignment and color. The block toolbar offers immediate options for block manipulation, including alignment and transformation. Beyond block settings, document settings help manage overall post or page setup, including categories and tags to organize content effectively.
Once your content is ready, options are available to save, preview, or publish it. The revision history feature lets you view previous versions. For further learning, official WordPress documentation and the Block Editor Handbook provide more in-depth guidance.
The WordPress Block Editor is a comprehensive tool that can enhance your content creation experience, making it vital to understand its functionalities to produce engaging, well-structured posts.