How to Debug a WordPress Website
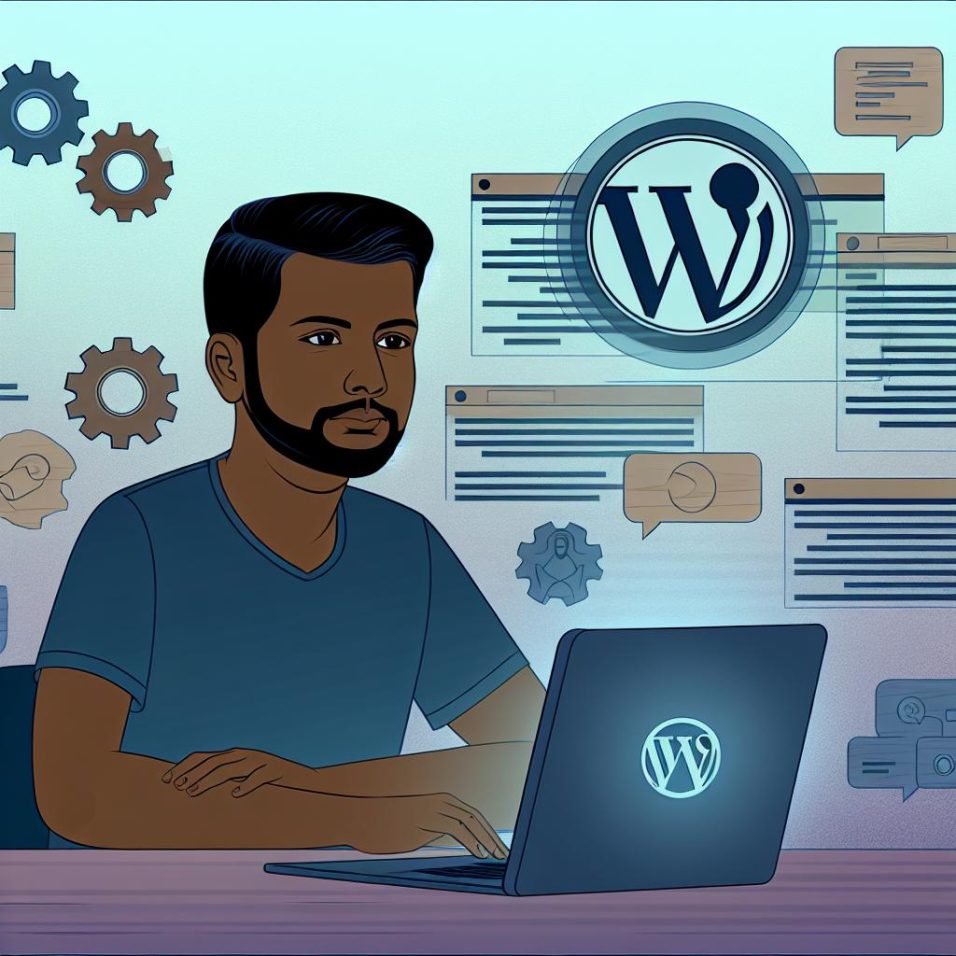
Debugging a WordPress site is crucial for maintaining its performance and security. It involves identifying and fixing errors to enhance user experience and prevent risks. This guide provides practical steps for debugging, suitable for both novices and seasoned developers.
**Enable WordPress Debugging Mode**
To initiate debugging, activate WordPress’s internal debugging feature via the `wp-config.php` file. Adding `define(‘WP_DEBUG’, true);` will display errors and notices, while `define(‘WP_DEBUG_LOG’, true);` logs errors to a file for production environments.
**Identify and Fix Common WordPress Errors**
Address frequent issues such as:
– **404 Errors on Posts**: Fix by resetting permalinks under Settings > Permalinks.
– **Internal Server Error (500)**: Resolve by renaming the `.htaccess` file or increasing PHP memory in `wp-config.php`.
**Deactivating Themes and Plugins**
Conflict between themes and plugins can cause problems. Diagnose by deactivating all plugins and reactivating them one by one, or switch to a default theme to identify the source.
**Examine Browser Console Errors**
Using browser developer tools can help spot JavaScript and server errors. This involves using the Console tab in the Inspect tool to find and address errors.
**Utilize Debugging Plugins**
Plugins like Query Monitor and WP Debugging provide insights into database queries and errors without editing code.
**Seek Assistance in WordPress Communities**
Engage with WordPress support forums or platforms like Stack Overflow for expert insights on complex issues.
Regular debugging ensures optimal WordPress site functionality and security. Embrace these methods and community support to enhance your troubleshooting prowess. Debugging might initially seem daunting, but with systematic troubleshooting, your site can swiftly regain optimal performance.